Here’s a quick PowerShell guide on how to store string values such as API keys in a text file so that any number of scripts used on the same system may access it securely.
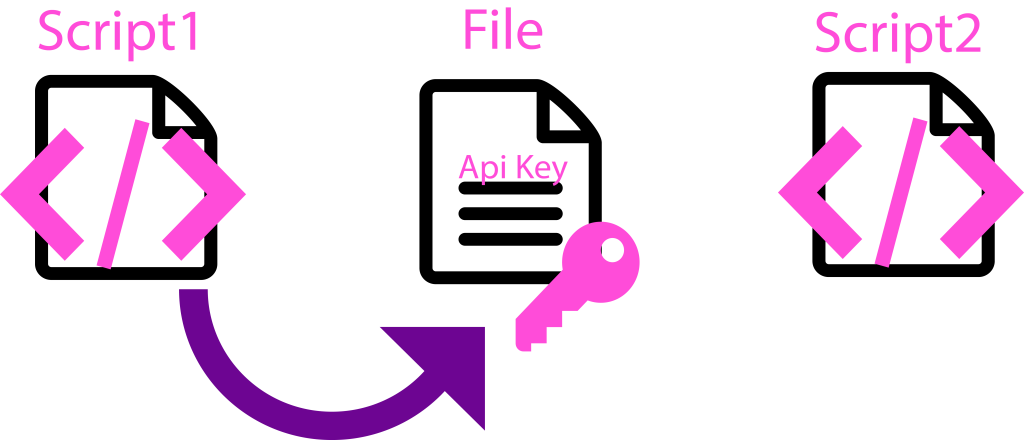
Quick Snippet
We have two cmdlets that we will be using: ConvertTo-SecureString & ConvertFrom-SecureString 1 . The first cmdlet will convert the string the user enters into a secure string. The second is used to convert to an encrypted string to be stored within the SecureApiKey.txt file.
Within Script 1
#Store Api credentials
$apiKey = Read-Host "Enter your API key"
$password = ConvertTo-SecureString $apiKey -AsPlainText -Force
$password | ConvertFrom-SecureString | Out-File ".\SecureApiKey.txt"
Once the text file is created, only the user that executed the ConvertFrom-SecureString may decrypt the string back to its original string value.
Within Script 2
#Retrieve Api credentials
$secureApiKey = Get-Content ".\SecureApiKey.txt" | ConvertTo-SecureString
$apiKey = [System.Runtime.InteropServices.Marshal]::PtrToStringAuto([System.Runtime.InteropServices.Marshal]::SecureStringToBSTR($secureApiKey))
ConvertTo-SecureString is used to convert the encrypted string back to a secure string and stored in the secureApiKey variable. Finally, the SecureStringtoBSTR and PtrToStringAuto from the Marshal class are used to convert the string back to plain text. 2 3
We have now successfully converted a plain text string into a secure string and back to plain text between 2 different scripts.
Process Explained
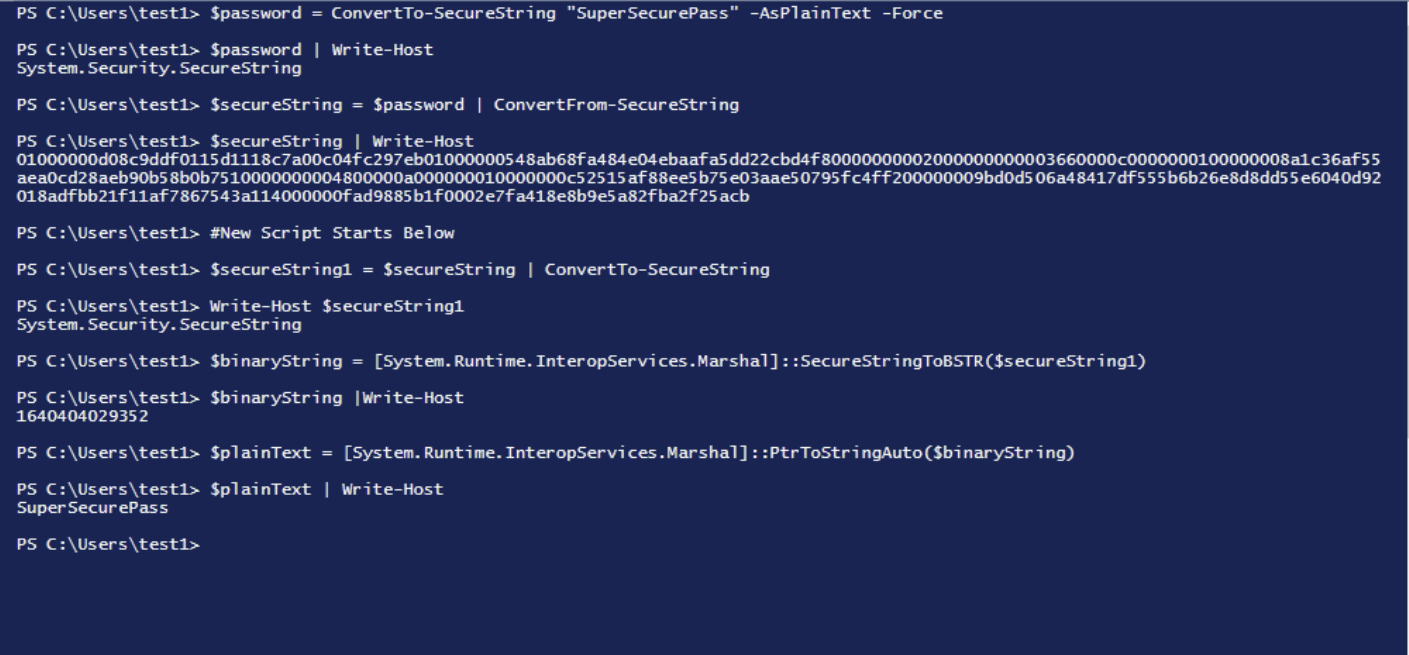
Encryption Process

1. The user is prompted for the plain text string .
2. The ConvertTo-SecureString takes the plain text and converts it to a system-secure string.
3. The ConvertFrom-SecureString takes the system secure string and converts it to an encrypted string using Microsoft’s Windows Data Protection API. 4
4. The text file stores the encrypted string that can only be decrypted using the key created by the Data Protection API.
Decryption Process

1. The text file with the encrypted string is retrieved
2. The ConvertTo-SecureString is used to convert the encrypted string to a system secure string
3. The SecureStringToBTSTR method from the .NET Marshal class is used to decrypt using the user’s encryption key and convert the system secure string to a binary string.
4. The PtrToStringAuto converts the binary string back to a regular string
# Programming